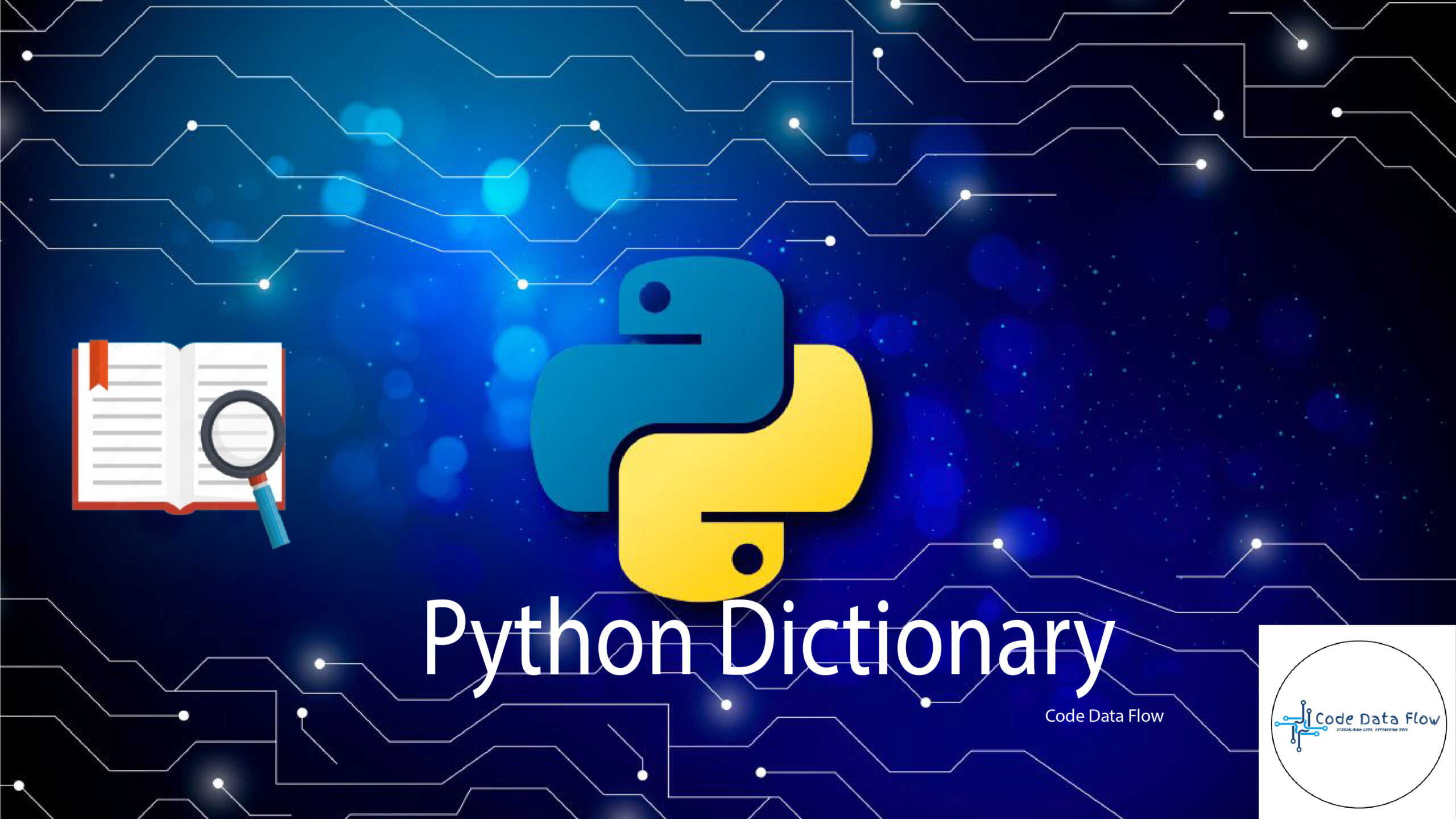
1.How to Use Dictionaries for Effective Data Structuring in Python
Course Overview: This course “How to Use Dictionaries for Effective Data Structuring in Python” will teach you about dictionaries in Python. You will learn how to use dictionaries for organizing, changing, and getting data. The course includes simple to advanced topics with coding examples and real-life situations.
Course Outline:
Module 1: Introduction to Dictionaries
Definition: This module talks about dictionaries in Python. It explains what they are, how they are not like lists and tuples, and when to use them.
- What is a Dictionary? A dictionary in Python is a collection of key-value pairs that is not ordered and can change. Each key is unique, linking to a particular value. Dictionaries are good for situations where you want to connect a unique key with some data.
- Key-Value Pairs Explained: A key-value pair is what makes up a dictionary. The key works as an identifier for a value, which helps in retrieving data quickly. For instance, in { “name”: “Alice” }, “name” is the key and “Alice” is the value.
- Differences in Lists, Tuples, and Dictionaries
- Lists: They are ordered and can be changed. You access items by their index.
- Tuples: They are ordered but cannot be changed. Accessing items is also done by index.
- Dictionaries: These are unordered and can be changed. You access items using keys.
- When to Use Dictionaries: Dictionaries are good when you want to match keys with values, find data fast, or keep track of settings. They work well for counting things, keeping data in order, and handling connections between items.
- Basic Syntax and Operations
- Creating a dictionary:
my_dict = {"key": "value"}
- Accessing values:
my_dict["key"]
- Adding/updating entries:
my_dict["new_key"] = "new_value"
- Deleting entries:
del my_dict["key"]
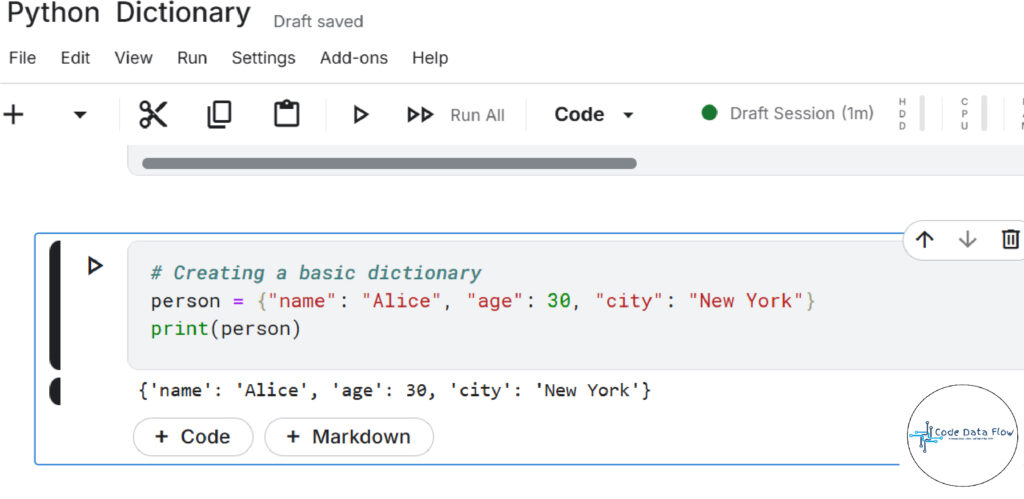
Module 2: Dictionary Operations
Definition:This module talks about basic tasks you can do with dictionaries, like adding, changing, getting rid of entries, and getting values.
- Adding, Changing, and Getting Rid of Key-Value Pairs Learn how to put in new entries, change old ones, and take away data that is not needed.
- Getting Values with Keys Get data easily using keys, and deal with missing keys in a good way.
- Checking if a Key Exists Use “in” to see if a key is in a dictionary before doing tasks.
- Using get() Method Get values safely with a backup return when keys are not there.
- Looping Through Dictionaries Look at different ways to go through keys, values, and key-value pairs.
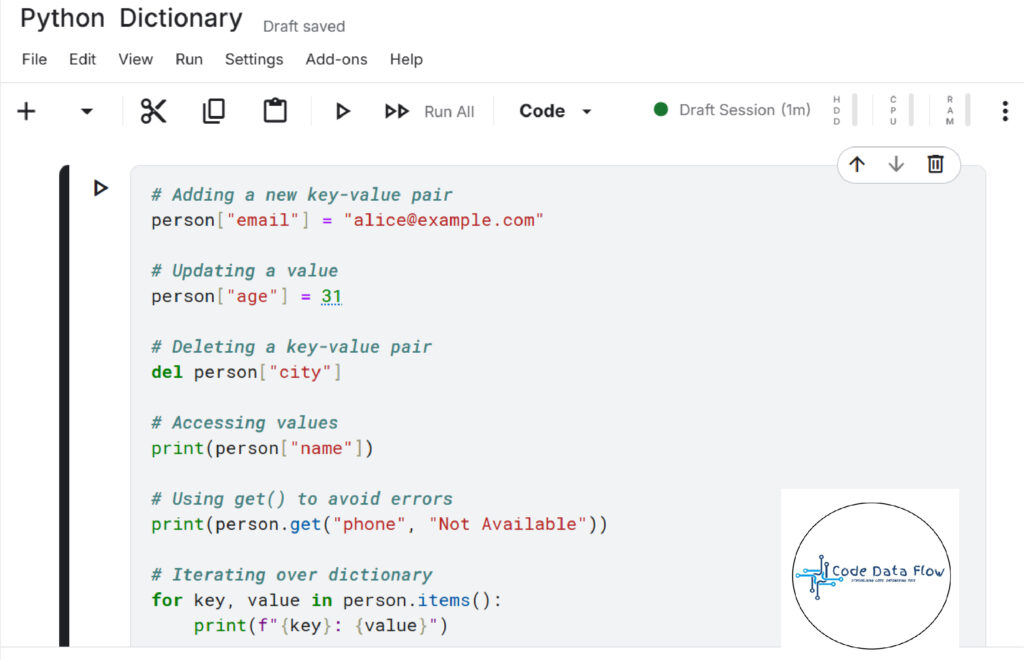
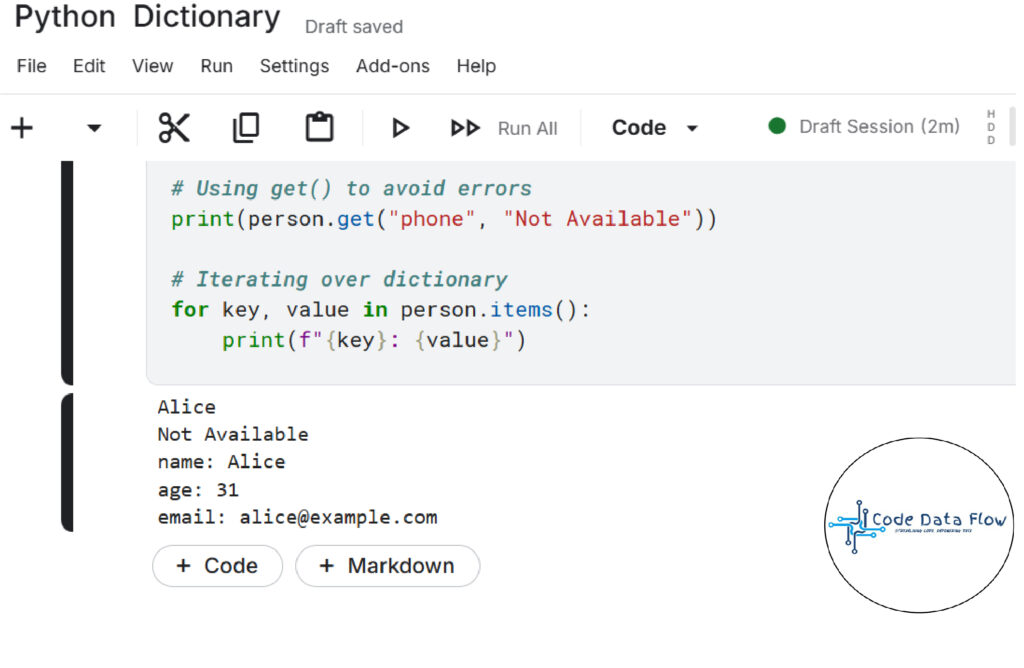
Module 3: Advanced Dictionary Concepts
Definition: Explore further advanced aspects of dictionaries, featuring nested dictionaries, comprehensions, and default values.
- Nested DictionariesUnderstand how to organize and oversee multi-layer data formats by utilizing dictionaries inside other dictionaries.
- Dictionary Comprehensions Generate dictionaries quickly and compactly through comprehension methods.
- Integrating Dictionaries with Loops and ConditionalsIncorporate dictionaries effectively with control statements for enhanced data processing.
- Combining and Modifying Dictionaries Join dictionaries effectively and manage duplicate keys.
- Using Default Values with defaultdict Make managing absent keys easier with the defaultdict available in the collections module.
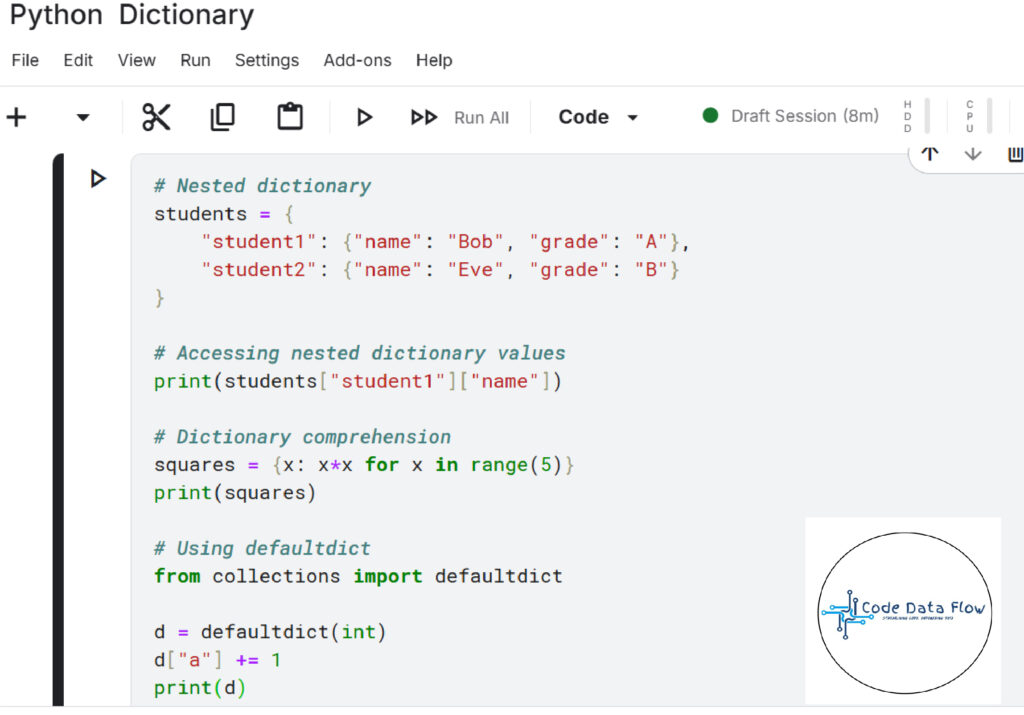
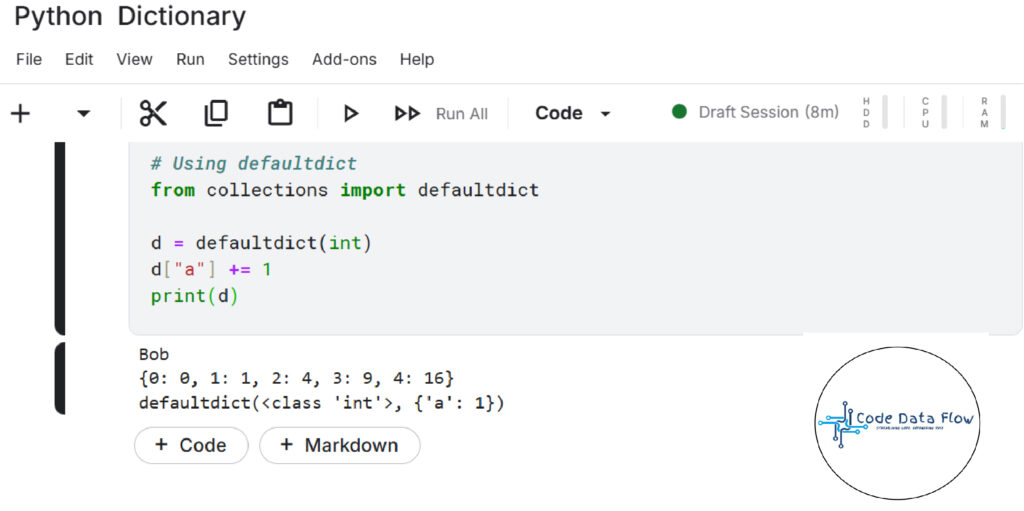
Module 4: Real-World Applications of Dictionaries
Definition: Learn to use dictionaries for solving problems in real life, like managing config settings and working with JSON data.
- Storing and Getting Configuration Settings Use dictionaries to manage application or script settings for simple access and changes.
- Counting Word Occurrences in a Text Utilize dictionaries to track how many times words appear in larger texts effectively.
- JSON Data Handling and Editing Understand how to load and modify JSON data using dictionaries to get information.
- Storing API Responses Manage data from APIs well by organizing replies as dictionaries.
- Creating Lookup Tables Create lookup tables that work well for fast data access using key-value pairs.
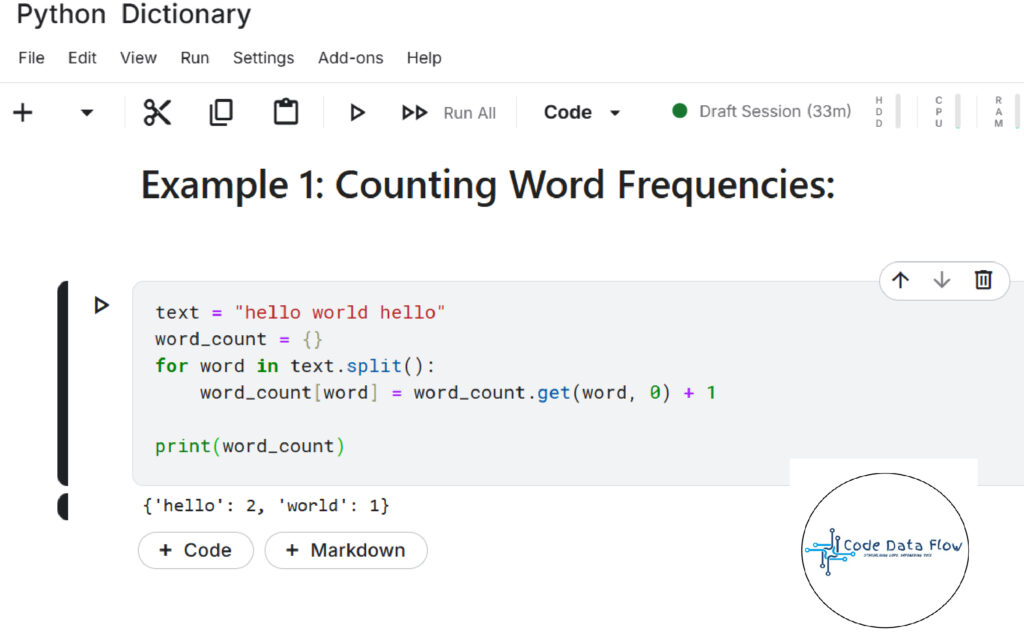
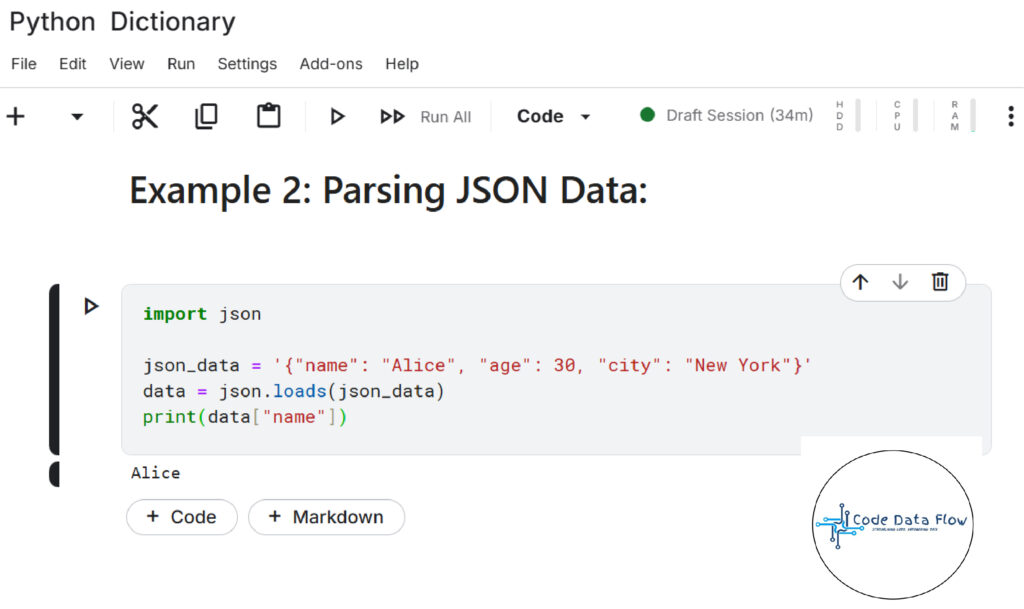
Module 5: Performance Optimization and Best Practices
Definition: This module looks at how well dictionaries work, focusing on improving performance, using memory, and best coding practices.
- Time Complexity of Dictionary Actions Understand how well common dictionary actions like searching, adding, and removing items perform.
- Memory Usage Points Learn how dictionaries use memory and how to improve for big data sets.
- Selecting the Good Data Structure Know when to use dictionaries instead of other structures.
- Typical Mistakes and How to Avoid Them Find common errors when using dictionaries and how to create solid code.
- Best Practices for Clear and Effective Code Stick to coding guidelines for clarity and efficiency when using dictionaries.
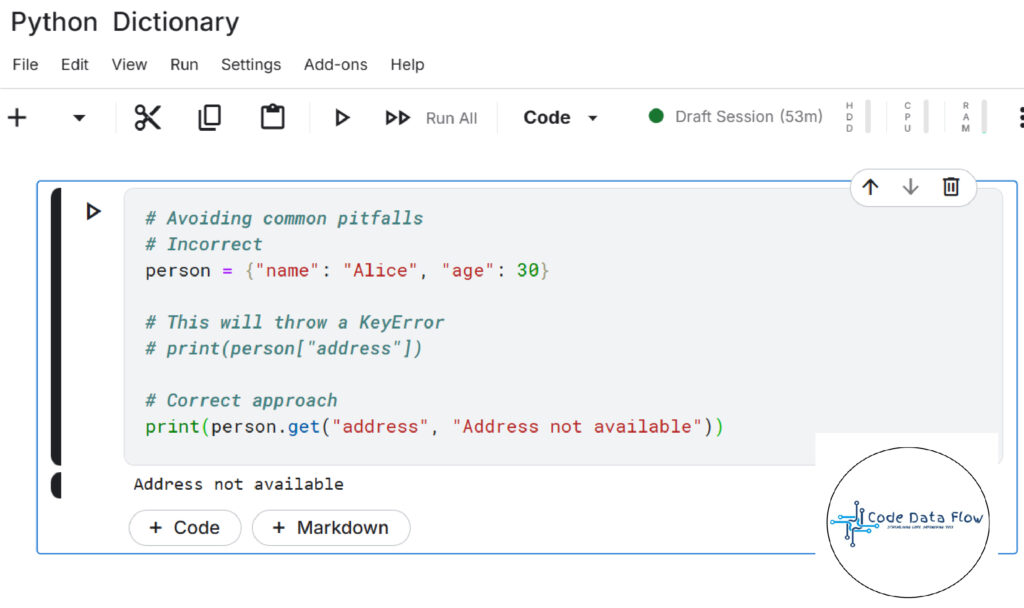
Module 6: Hands-On Projects
Definition: Apply what you’ve learned in practical, hands-on projects to reinforce your understanding of dictionaries.
Store and Retrieve Student Grades, Calculate Averages
Project 1: Contact Book Application
Create, Update, Search, and Delete Contacts using Dictionaries
Project 2: Word Frequency Counter
Analyze and Display Word Frequencies from a Text File
Project 3: API Data Handling
Fetch and Structure API Data Using Dictionaries
Project 4: Student Grade Management System
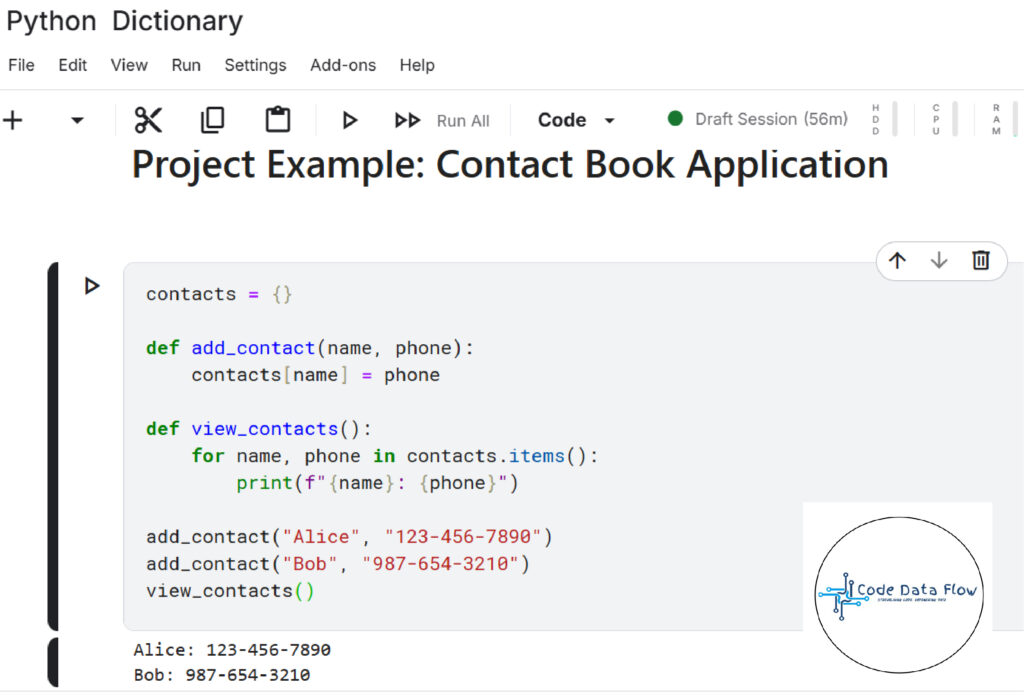
Conclusion: At the end of this course, you will know how to use dictionaries for data organization in Python. You will see how to use these ideas in real situations, making your coding better and helping with solving problems.