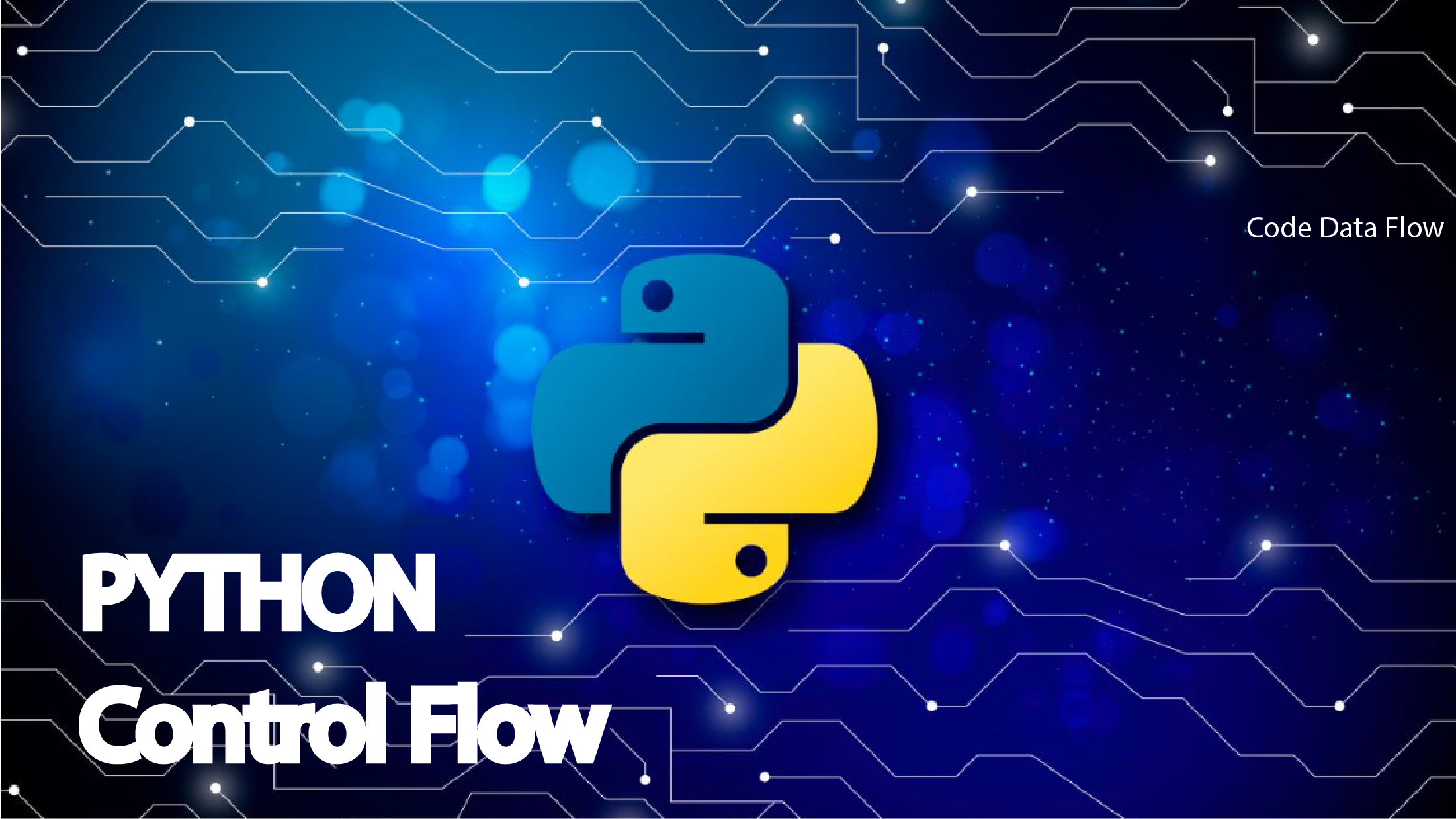
Learn Python Control Flow
Lecture 1.3: Learn Control Flow in Python
Introduction to Control Flow in Python
Control flow allows you to write code that performs specific actions depending on various conditions. Python provides several control flow mechanisms, such as conditional statements (if
, elif
, else
), loops (for
, while
), and more advanced tools like break
, continue
, and pass
. In this lecture, we will cover how control flow structures are used to control the flow of execution in a Python program.
1. Conditional Statements: if
, elif
, else
:
Conditional statements are used to perform different actions based on different conditions. Python uses if
, elif
, and else
to write decision-making code.
Syntax of Conditional Statements
if condition:
# Code block to execute if condition is true
elif another_condition:
# Code block to execute if the above condition is false but this one is true
else:
# Code block to execute if none of the conditions are true
Example:
x = 10
if x > 10:
print("x is greater than 10")
elif x == 10:
print("x is equal to 10")
else:
print("x is less than 10")
2. Loops in Python: for
and while
:
Loops are used to iterate over a sequence (like a list, tuple, dictionary, string) or execute a block of code repeatedly until a condition is met.
2.1 The for
Loop
A for
loop is used to iterate over a sequence. You can loop through the elements of a list, string, or any iterable.
Syntax:
for item in sequence:
# Code block to execute
Example:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
2.2 The while
Loop
The while
loop continues to execute as long as a given condition is True
.
Syntax:
while condition:
# Code block to execute
Example:
count = 0
while count < 5:
print(count)
count += 1 # Increment count
3. Control Flow Tools: break
, continue
, and pass
:
Python provides special keywords like break
, continue
, and pass
to alter the flow of a loop.
3.1 The break
Statement
break
is used to terminate the loop prematurely when a certain condition is met.
Example:
for num in range(10):
if num == 5:
break # Exit loop when num is 5
print(num)
3.2 The continue
Statement
continue
skips the current iteration and moves to the next iteration of the loop.
Example:
for num in range(5):
if num == 3:
continue # Skip the iteration when num is 3
print(num)
3.3 The pass
Statement
pass
does nothing; it is used when a statement is syntactically required but you don’t want to execute any code.
Example:
for num in range(5):
if num == 3:
pass # Do nothing when num is 3
print(num)
4. Nested Conditional Statements and Loops:
You can nest if
statements and loops inside one another to handle more complex conditions and repetitions.
Example of Nested if
Statements:
x = 15
if x > 10:
if x < 20:
print("x is between 10 and 20")
Example of Nested Loops:
for i in range(3):
for j in range(3):
print(i, j)
5. The else
Clause with Loops:
Python allows you to use an else
clause with loops. The else
part is executed after the loop finishes, unless the loop is terminated by a break
.
Example:
for num in range(5):
print(num)
else:
print("Loop completed")
6. Exception Handling:
In some cases, errors occur during program execution. Python uses try
, except
, and finally
to handle exceptions and errors gracefully.
Example:
try:
result = 10 / 0 # This will raise a division by zero error
except ZeroDivisionError:
print("Cannot divide by zero!")
finally:
print("This block runs no matter what")
Conclusion:
Control flow is a fundamental concept in Python programming that enables the decision-making process and repetition of code blocks. By mastering conditional statements, loops, and control flow tools, you can write more efficient and logical programs. This foundation will be essential as you advance in Python for data science and tackle more complex problems like data manipulation, analysis, and machine learning.